- ManualResetEvent
- AutoResetEvent
- AutoResetEvent 클래스 (http://www.csharpstudy.com)
- ManualResetEvent 클래스 (http://www.csharpstudy.com)
- CountdownEvent 클래스 (http://www.csharpstudy.com)
AutoResetEvent
1. Set() 을 호출하면 Wait 하고 있는 쓰레드를 중에서 처음 한개만 통과시키고 나머지는 대기시킴
2. 다시 Set() 호출하면 그 다음 쓰레드를 통과시키고 나머지는 대기시킴.
ManualResetEvent
1. Set() 을 호출하면 Wait 하고 있는 쓰레드 모두 통과시킴. (계속 통과 상태)
2. Reset() 을 호출하면 다시 대기 상태
ManualResetEvent Class
https://msdn.microsoft.com/en-us/library/system.threading.manualresetevent(v=vs.110).aspx
Notifies one or more waiting threads that an event has occurred. This class cannot be inherited.
Namespace: System.Threading
Assembly: mscorlib (in mscorlib.dll)
Inheritance Hierarchy
System.MarshalByRefObject
System.Threading.WaitHandle
System.Threading.EventWaitHandle
System.Threading.ManualResetEvent
Remarks
In the .NET Framework version 2.0, ManualResetEvent derives from the new EventWaitHandle class. A ManualResetEvent is functionally equivalent to an EventWaitHandle created with EventResetMode.ManualReset.
![]() |
---|
Unlike the ManualResetEvent class, the EventWaitHandle class provides access to named system synchronization events. |
ManualResetEvent allows threads to communicate with each other by signaling. Typically, this communication concerns a task which one thread must complete before other threads can proceed.
When a thread begins an activity that must complete before other threads proceed, it calls Reset to put ManualResetEvent in the non-signaled state. This thread can be thought of as controlling the ManualResetEvent. Threads that call WaitOne on the ManualResetEvent will block, awaiting the signal. When the controlling thread completes the activity, it calls Set to signal that the waiting threads can proceed. All waiting threads are released.
Once it has been signaled, ManualResetEvent remains signaled until it is manually reset. That is, calls to WaitOne return immediately.
You can control the initial state of a ManualResetEvent by passing a Boolean value to the constructor, true if the initial state is signaled and false otherwise.
ManualResetEvent can also be used with the staticWaitAll and WaitAny methods.
For more information about thread synchronization mechanisms, see ManualResetEvent and ManualResetEventSlim in the conceptual documentation.
Examples
The following example demonstrates how ManualResetEvent works. The example starts with a ManualResetEvent in the unsignaled state (that is, false is passed to the constructor). The example creates three threads, each of which blocks on the ManualResetEvent by calling its WaitOne method. When the user presses the Enter key, the example calls the Set method, which releases all three threads. Contrast this with the behavior of the AutoResetEvent class, which releases threads one at a time, resetting automatically after each release.
Pressing the Enter key again demonstrates that the ManualResetEvent remains in the signaled state until its Reset method is called: The example starts two more threads. These threads do not block when they call the WaitOne method, but instead run to completion.
Pressing the Enter key again causes the example to call the Reset method and to start one more thread, which blocks when it calls WaitOne. Pressing the Enter key one final time calls Set to release the last thread, and the program ends.
using System; using System.Threading; public class Example { // mre is used to block and release threads manually. It is // created in the unsignaled state. private static ManualResetEvent mre = new ManualResetEvent(false); static void Main() { Console.WriteLine("\nStart 3 named threads that block on a ManualResetEvent:\n"); for(int i = 0; i <= 2; i++) { Thread t = new Thread(ThreadProc); t.Name = "Thread_" + i; t.Start(); } Thread.Sleep(500); Console.WriteLine("\nWhen all three threads have started, press Enter to call Set()" + "\nto release all the threads.\n"); Console.ReadLine(); mre.Set(); Thread.Sleep(500); Console.WriteLine("\nWhen a ManualResetEvent is signaled, threads that call WaitOne()" + "\ndo not block. Press Enter to show this.\n"); Console.ReadLine(); for(int i = 3; i <= 4; i++) { Thread t = new Thread(ThreadProc); t.Name = "Thread_" + i; t.Start(); } Thread.Sleep(500); Console.WriteLine("\nPress Enter to call Reset(), so that threads once again block" + "\nwhen they call WaitOne().\n"); Console.ReadLine(); mre.Reset(); // Start a thread that waits on the ManualResetEvent. Thread t5 = new Thread(ThreadProc); t5.Name = "Thread_5"; t5.Start(); Thread.Sleep(500); Console.WriteLine("\nPress Enter to call Set() and conclude the demo."); Console.ReadLine(); mre.Set(); // If you run this example in Visual Studio, uncomment the following line: //Console.ReadLine(); } private static void ThreadProc() { string name = Thread.CurrentThread.Name; Console.WriteLine(name + " starts and calls mre.WaitOne()"); mre.WaitOne(); Console.WriteLine(name + " ends."); } } /* This example produces output similar to the following: Start 3 named threads that block on a ManualResetEvent: Thread_0 starts and calls mre.WaitOne() Thread_1 starts and calls mre.WaitOne() Thread_2 starts and calls mre.WaitOne() When all three threads have started, press Enter to call Set() to release all the threads. Thread_2 ends. Thread_0 ends. Thread_1 ends. When a ManualResetEvent is signaled, threads that call WaitOne() do not block. Press Enter to show this. Thread_3 starts and calls mre.WaitOne() Thread_3 ends. Thread_4 starts and calls mre.WaitOne() Thread_4 ends. Press Enter to call Reset(), so that threads once again block when they call WaitOne(). Thread_5 starts and calls mre.WaitOne() Press Enter to call Set() and conclude the demo. Thread_5 ends. */
AutoResetEvent
https://msdn.microsoft.com/en-us/library/system.threading.autoresetevent(v=vs.110).aspx
Notifies a waiting thread that an event has occurred. This class cannot be inherited.
Namespace: System.ThreadingAssembly: mscorlib (in mscorlib.dll)
Inheritance Hierarchy
System.MarshalByRefObject
System.Threading.WaitHandle
System.Threading.EventWaitHandle
System.Threading.AutoResetEvent
Remarks
AutoResetEvent allows threads to communicate with each other by signaling. Typically, you use this class when threads need exclusive access to a resource.
![]() |
---|
This type implements the IDisposable interface. When you have finished using the type, you should dispose of it either directly or indirectly. To dispose of the type directly, call its Dispose method in a try/catch block. To dispose of it indirectly, use a language construct such as using (in C#) or Using (in Visual Basic). For more information, see the “Using an Object that Implements IDisposable” section in the IDisposable interface topic. |
A thread waits for a signal by calling WaitOne on the AutoResetEvent. If the AutoResetEvent is in the non-signaled state, the thread blocks, waiting for the thread that currently controls the resource to signal that the resource is available by calling Set.
Calling Set signals AutoResetEvent to release a waiting thread. AutoResetEvent remains signaled until a single waiting thread is released, and then automatically returns to the non-signaled state. If no threads are waiting, the state remains signaled indefinitely.
If a thread calls WaitOne while the AutoResetEvent is in the signaled state, the thread does not block. The AutoResetEvent releases the thread immediately and returns to the non-signaled state.
![]() |
---|
There is no guarantee that every call to the Set method will release a thread. If two calls are too close together, so that the second call occurs before a thread has been released, only one thread is released. It is as if the second call did not happen. Also, if Set is called when there are no threads waiting and the AutoResetEvent is already signaled, the call has no effect. |
You can control the initial state of an AutoResetEvent by passing a Boolean value to the constructor: true if the initial state is signaled and false otherwise.
AutoResetEvent can also be used with the staticWaitAll and WaitAny methods.
For more information about thread synchronization mechanisms, see AutoResetEvent in the conceptual documentation.
Beginning with the .NET Framework version 2.0, AutoResetEvent derives from the new EventWaitHandle class. An AutoResetEvent is functionally equivalent to an EventWaitHandle created with EventResetMode.AutoReset.
![]() |
---|
Unlike the AutoResetEvent class, the EventWaitHandle class provides access to named system synchronization events. |
Examples
The following example shows how to use AutoResetEvent to release one thread at a time, by calling the Set method (on the base class) each time the user presses the Enter key. The example starts three threads, which wait on an AutoResetEvent that was created in the signaled state. The first thread is released immediately, because the AutoResetEvent is already in the signaled state. This resets the AutoResetEvent to the non-signaled state, so that subsequent threads block. The blocked threads are not released until the user releases them one at a time by pressing the Enter key.
After the threads are released from the first AutoResetEvent, they wait on another AutoResetEvent that was created in the non-signaled state. All three threads block, so the Set method must be called three times to release them all.
using System; using System.Threading; // Visual Studio: Replace the default class in a Console project with // the following class. class Example { private static AutoResetEvent event_1 = new AutoResetEvent(true); private static AutoResetEvent event_2 = new AutoResetEvent(false); static void Main() { Console.WriteLine("Press Enter to create three threads and start them.\r\n" + "The threads wait on AutoResetEvent #1, which was created\r\n" + "in the signaled state, so the first thread is released.\r\n" + "This puts AutoResetEvent #1 into the unsignaled state."); Console.ReadLine(); for (int i = 1; i < 4; i++) { Thread t = new Thread(ThreadProc); t.Name = "Thread_" + i; t.Start(); } Thread.Sleep(250); for (int i = 0; i < 2; i++) { Console.WriteLine("Press Enter to release another thread."); Console.ReadLine(); event_1.Set(); Thread.Sleep(250); } Console.WriteLine("\r\nAll threads are now waiting on AutoResetEvent #2."); for (int i = 0; i < 3; i++) { Console.WriteLine("Press Enter to release a thread."); Console.ReadLine(); event_2.Set(); Thread.Sleep(250); } // Visual Studio: Uncomment the following line. //Console.Readline(); } static void ThreadProc() { string name = Thread.CurrentThread.Name; Console.WriteLine("{0} waits on AutoResetEvent #1.", name); event_1.WaitOne(); Console.WriteLine("{0} is released from AutoResetEvent #1.", name); Console.WriteLine("{0} waits on AutoResetEvent #2.", name); event_2.WaitOne(); Console.WriteLine("{0} is released from AutoResetEvent #2.", name); Console.WriteLine("{0} ends.", name); } } /* This example produces output similar to the following: Press Enter to create three threads and start them. The threads wait on AutoResetEvent #1, which was created in the signaled state, so the first thread is released. This puts AutoResetEvent #1 into the unsignaled state. Thread_1 waits on AutoResetEvent #1. Thread_1 is released from AutoResetEvent #1. Thread_1 waits on AutoResetEvent #2. Thread_3 waits on AutoResetEvent #1. Thread_2 waits on AutoResetEvent #1. Press Enter to release another thread. Thread_3 is released from AutoResetEvent #1. Thread_3 waits on AutoResetEvent #2. Press Enter to release another thread. Thread_2 is released from AutoResetEvent #1. Thread_2 waits on AutoResetEvent #2. All threads are now waiting on AutoResetEvent #2. Press Enter to release a thread. Thread_2 is released from AutoResetEvent #2. Thread_2 ends. Press Enter to release a thread. Thread_1 is released from AutoResetEvent #2. Thread_1 ends. Press Enter to release a thread. Thread_3 is released from AutoResetEvent #2. Thread_3 ends. */
1. signaled 상태에서는 Set() 호출해도 non-signaled로 안됨
2. non-signaled 상태에서는 Set() 호출하면 signaled로 됨
AutoResetEvent 클래스
http://www.csharpstudy.com/Threads/autoresetevent.aspx
예제
예제
ManualResetEvent 클래스
http://www.csharpstudy.com/Threads/manualresetevent.aspx
예제
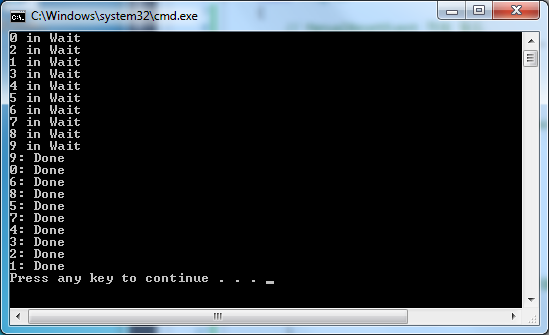
CountdownEvent 클래스
http://www.csharpstudy.com/Threads/countdownevent.aspx
예제
'프로그래밍 > C#' 카테고리의 다른 글
Events (0) | 2017.03.29 |
---|---|
Common I/O Tasks (0) | 2017.03.15 |
Sockets (0) | 2017.03.02 |
Using the Visual Studio Development Environment for C# (0) | 2017.02.14 |
Getting Started with C# (0) | 2017.02.13 |